The Easiest Computational Fluid Dynamics Software
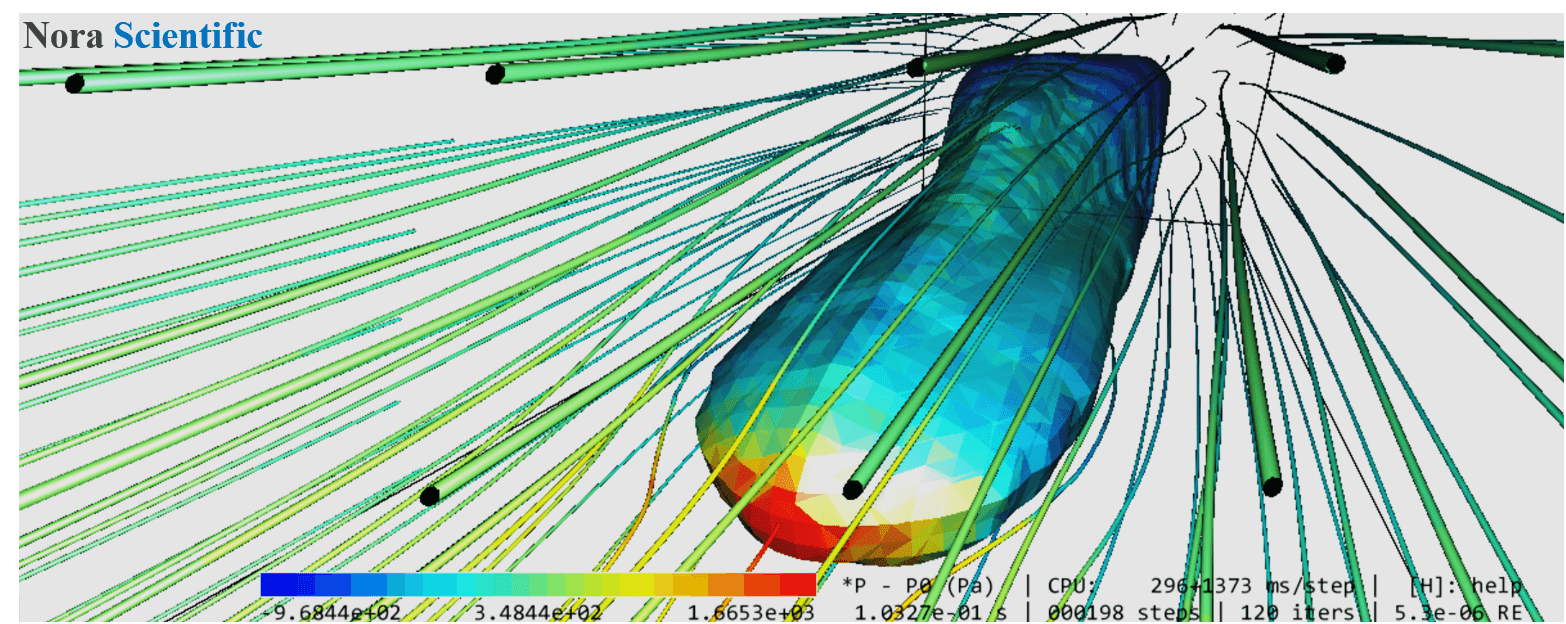
Solution Data Files
File Contents
During a simulation in Flowsquare+, instantaneous solution data are saved once in every nfile time steps in the ./dump directory in a project folder. These instantaneous data consist of following files contained in a folder named according to time step that the data were saved.
- info.txt
This text file contains basic simulation information such as physical time, time step size, number of mesh points. - ufld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of X-direction velocity component (U). - vfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of Y-direction velocity component (V). - wfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of Z-direction velocity component (W). - yfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of mass fraction of substance in the fluid. - afld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of fluid age variable. - pfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of pressure (p-presW). - rfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of fluid density (only for cmode=1). - tfld.raw
3D binary data with dimensions of (nx, ny, nz) in double precision of temperature (only for cmode=1).
These bindary files can be loaded in Flowsquare+ after simulation using the "analysis mode", to visualize and for further analysis. They can be also visualized by using any binary data visualization tool such as Paraview.
In the above binary files, the simulation domain of (lx, ly, lz) is discretised by (nx, ny, nz) of uniform mesh points, and their mesh size for x, y, z directions can be calculated respectively as lx/(nx-1), ly/(ny-1) and lz/(nz-1).
Here is a Python script to load dump data, get a field value at arbitorary physical (x,y,z) and mesh (i,j,k) location, and to save a XY plane at k in a csv format. The values in the below script are based on the default case.
import numpy as np filepath="./ufld.raw" # from parameter nx = 60 ny = 30 nz = 30 lx = 10.0 ly = 5.0 lz = 5.0 dx = lx / float(nx-1) dy = ly / float(ny-1) dz = lz / float(nz-1) def load_field(): try: with open(filepath, "rb") as f: return np.fromfile(f,dtype='float') except: print('File not found at "'+filepath + '"') def save_xy_plane_csv(k, field, csv_filepath): # save an xy plane at k in a csv format. xy = field[nx*ny*k:nx*ny*(k+1)].reshape(ny,nx) np.savetxt(csv_filepath, np.flipud(xy), delimiter=',', fmt='%.5e') def get_value_at_xyz(x,y,z,field): # get a value at nearest mesh point from (x,y,z) on the field. if x<0 or y<0 or z<0 or x>lx or y>ly or z>lz: return -1 i = int(x / dx) j = int(y / dy) k = int(z / dz) return field[i + nx * (j + ny * k)] def get_value_at_ijk(i,j,k,field): # get a value at (i,j,k). if i<0 or j<0 or k<0 or i>nx-1 or j>ny-1 or k>nz-1: return -1 return field[i + nx * (j + ny * k)] field = load_field() print(get_value_at_xyz(10.0,0,0,field)) print(get_value_at_ijk(10,0,0,field)) save_xy_plane_csv(14, field, './test.csv')